Golang Int to String: Master Conversion Effortlessly
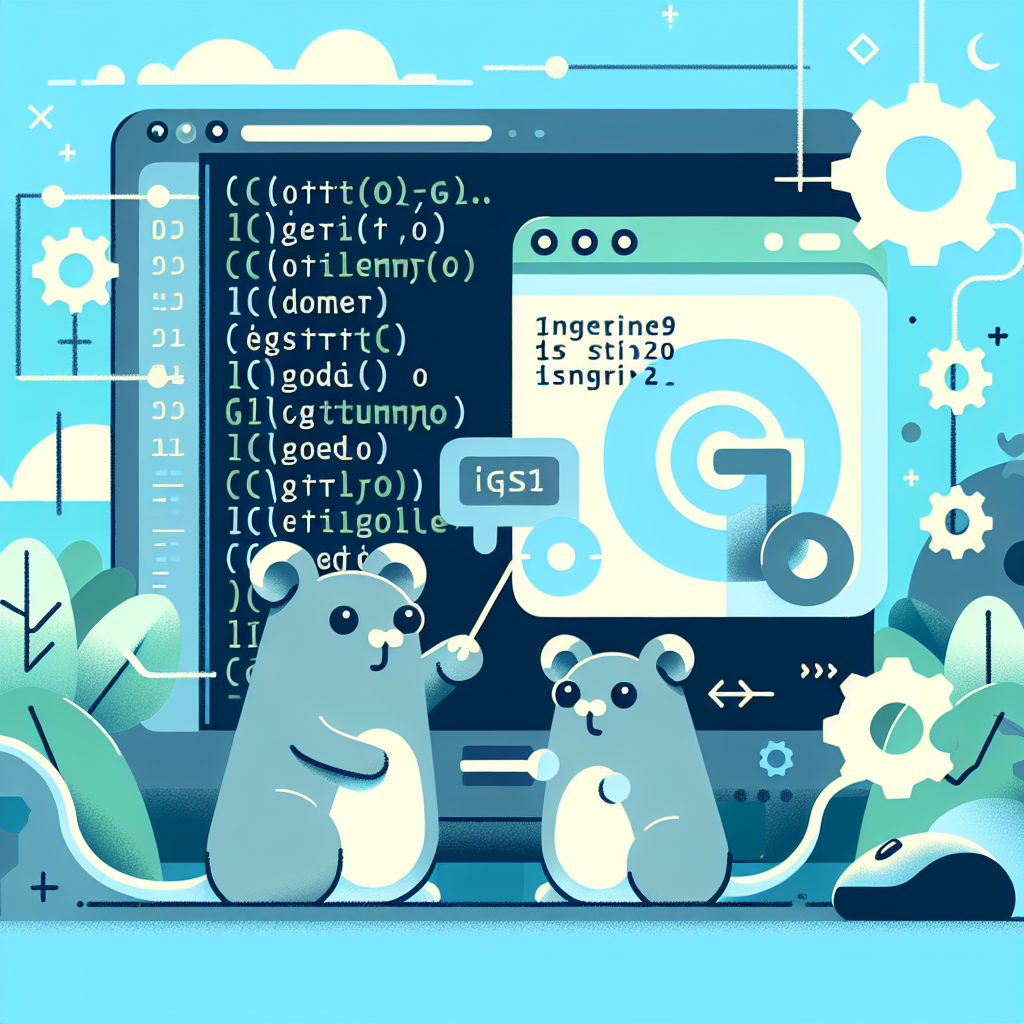
Introduction
Golang, commonly known as Go, is a statically typed, compiled programming language designed for simplicity and efficiency. One common task in Go programming is converting data types, such as turning an integer into a string. This process, known as “Golang int to string” conversion, is crucial for formatting output, generating user-friendly messages, and storing data in text-based formats. In this blog post, we’ll explore various methods for integer to string conversion in Go, enhance your understanding of Go programming, and provide practical tips to improve your coding skills.
Understanding Golang Conversion
In Go, converting an integer to a string is a straightforward task, but choosing the right method depends on your specific requirements. Here are some of the most common methods to perform this conversion:
Using strconv.Itoa
The strconv
package in Go provides a function called Itoa
, which converts an integer to a decimal string.
package main
import (
"fmt"
"strconv"
)
func main() {
num := 42
str := strconv.Itoa(num)
fmt.Println("Converted string:", str)
}
- Pros: Simple and direct method for converting integers to strings.
- Cons: Limited to base 10 conversions.
Using fmt.Sprintf
Another versatile method is using fmt.Sprintf
, which formats according to a format specifier.
package main
import (
"fmt"
)
func main() {
num := 42
str := fmt.Sprintf("%d", num)
fmt.Println("Formatted string:", str)
}
- Pros: Flexible and allows formatting of multiple data types.
- Cons: Slightly less performant due to its general-purpose nature.
Using strconv.FormatInt
The strconv.FormatInt
function allows you to specify the base of the conversion, offering more flexibility.
package main
import (
"fmt"
"strconv"
)
func main() {
num := int64(42)
str := strconv.FormatInt(num, 10)
fmt.Println("Base 10 string:", str)
}
- Pros: Supports different numeral systems (binary, octal, hexadecimal).
- Cons: Requires conversion to
int64
for compatibility.
Go Programming Tips for Efficient Conversion
To optimize your code and ensure efficient Golang conversion, consider the following tips:
Choosing the Right Method
- Simple Needs: Use
strconv.Itoa
for straightforward base 10 conversions. - Complex Formatting: Opt for
fmt.Sprintf
when you need to format multiple variables or include additional text. - Base Variations: Use
strconv.FormatInt
when working with different numeral systems.
Performance Considerations
- Benchmarking: Use Go’s benchmarking tools to compare the performance of different conversion methods in your specific use case.
- Avoid Unnecessary Conversions: If you’re repeatedly converting the same integer, store the result to avoid redundant operations.
Practical Examples and Use Cases
Example 1: Logging Integer Values
When logging numeric values, converting them to strings can make the logs more readable and easier to search.
package main
import (
"fmt"
"strconv"
)
func logValue(value int) {
logMessage := "The value is: " + strconv.Itoa(value)
fmt.Println(logMessage)
}
func main() {
logValue(100)
}
Example 2: Generating User-Friendly Output
In user-facing applications, converting numbers to strings can make interfaces more intuitive.
package main
import (
"fmt"
)
func formatPrice(price int) string {
return fmt.Sprintf("$%d", price)
}
func main() {
fmt.Println("Total Price:", formatPrice(199))
}
Conclusion
Converting an integer to a string in Golang is a fundamental task that can be accomplished through various methods. By understanding and choosing the appropriate conversion technique, you can write cleaner, more efficient Go code. Whether you’re logging data, formatting output, or working with different numeral systems, mastering Golang conversion will enhance your programming skills and lead to more effective code. Keep experimenting with different approaches and incorporate these Go programming tips to elevate your development practices.