Master Python Array Slice: Unlock Efficient Coding Today
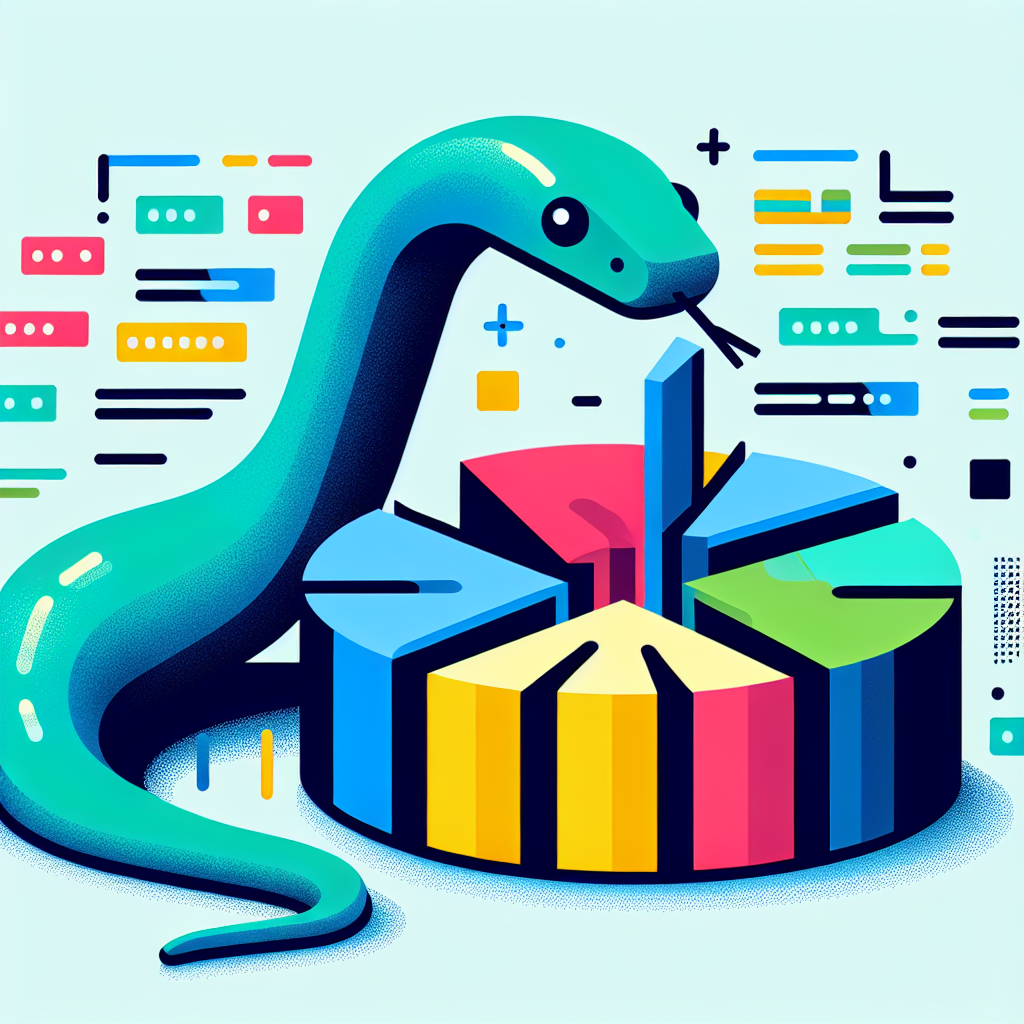
Introduction to Python Array Slice
Python is renowned for its simplicity and powerful features, one of which is array slicing. The Python array slice is an integral part of Python list manipulation, enabling developers to access specific parts of a list or array efficiently. Understanding how to leverage this feature can optimize your code and enhance its readability.
Python slicing is an essential skill for any Python programmer. It allows you to extract parts of sequences such as lists, tuples, and strings, making it a cornerstone of Python’s data manipulation capabilities.
Understanding Python Slicing
What is Python Slicing?
Python slicing involves accessing a range of elements from a sequence using a special syntax. This is done using the colon :
operator, which allows you to specify a start, stop, and step value.
The basic syntax for Python slicing is:
sequence[start:stop:step]
- start: The index where the slice starts (inclusive).
- stop: The index where the slice ends (exclusive).
- step: The interval between elements in the slice.
Python Slicing Tutorial
Let’s break down the slicing syntax with examples:
-
Basic Slicing:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] slice_1 = numbers[2:5] print(slice_1) # Output: [2, 3, 4]
-
Slicing with Step:
slice_2 = numbers[1:8:2] print(slice_2) # Output: [1, 3, 5, 7]
-
Omitting Parameters:
- If you omit the start, it defaults to the beginning of the list.
- If you omit the stop, it defaults to the end of the list.
- If you omit the step, it defaults to 1.
slice_3 = numbers[:4] print(slice_3) # Output: [0, 1, 2, 3] slice_4 = numbers[5:] print(slice_4) # Output: [5, 6, 7, 8, 9] slice_5 = numbers[::3] print(slice_5) # Output: [0, 3, 6, 9]
Advanced Array Slicing Examples
Negative Indexing
Python supports negative indexing, which allows you to slice from the end of the list.
reverse_slice = numbers[-3:]
print(reverse_slice) # Output: [7, 8, 9]
Reversing a List
You can reverse a list using slicing by setting the step to -1
.
reversed_list = numbers[::-1]
print(reversed_list) # Output: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
Multi-level Slicing
When working with multi-dimensional arrays (like lists of lists), slicing becomes slightly more complex.
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
row_slice = matrix[:2] # Slices the first two rows
print(row_slice) # Output: [[1, 2, 3], [4, 5, 6]]
column_slice = [row[1] for row in matrix] # Extracts the second column
print(column_slice) # Output: [2, 5, 8]
Practical Applications of Python Slicing
Python slicing is not just for lists; it extends to tuples and strings, offering versatility in data manipulation.
Slicing Strings
Strings in Python can be sliced just like lists.
text = "Python is fun!"
substring = text[7:9]
print(substring) # Output: "is"
Efficient Data Manipulation
Slicing is computationally efficient and helps in tasks like:
- Data Analysis: Extract subsets of data for analysis.
- Data Cleaning: Remove unwanted sections of data.
- Algorithm Optimization: Simplify and speed up processes by narrowing down datasets.
Conclusion
Mastering Python array slice is a valuable skill for any programmer. It enhances your ability to manipulate data efficiently, making your code cleaner and more readable. Whether you’re slicing lists, tuples, or strings, the principles remain the same, providing consistency across different data types.
The versatility of Python slicing makes it a powerful tool in any developer’s toolkit. By understanding and applying array slicing examples, you can elevate your Python list manipulation capabilities to new heights. Python’s slicing feature, with its simplicity and power, truly empowers developers to write better, more efficient code.