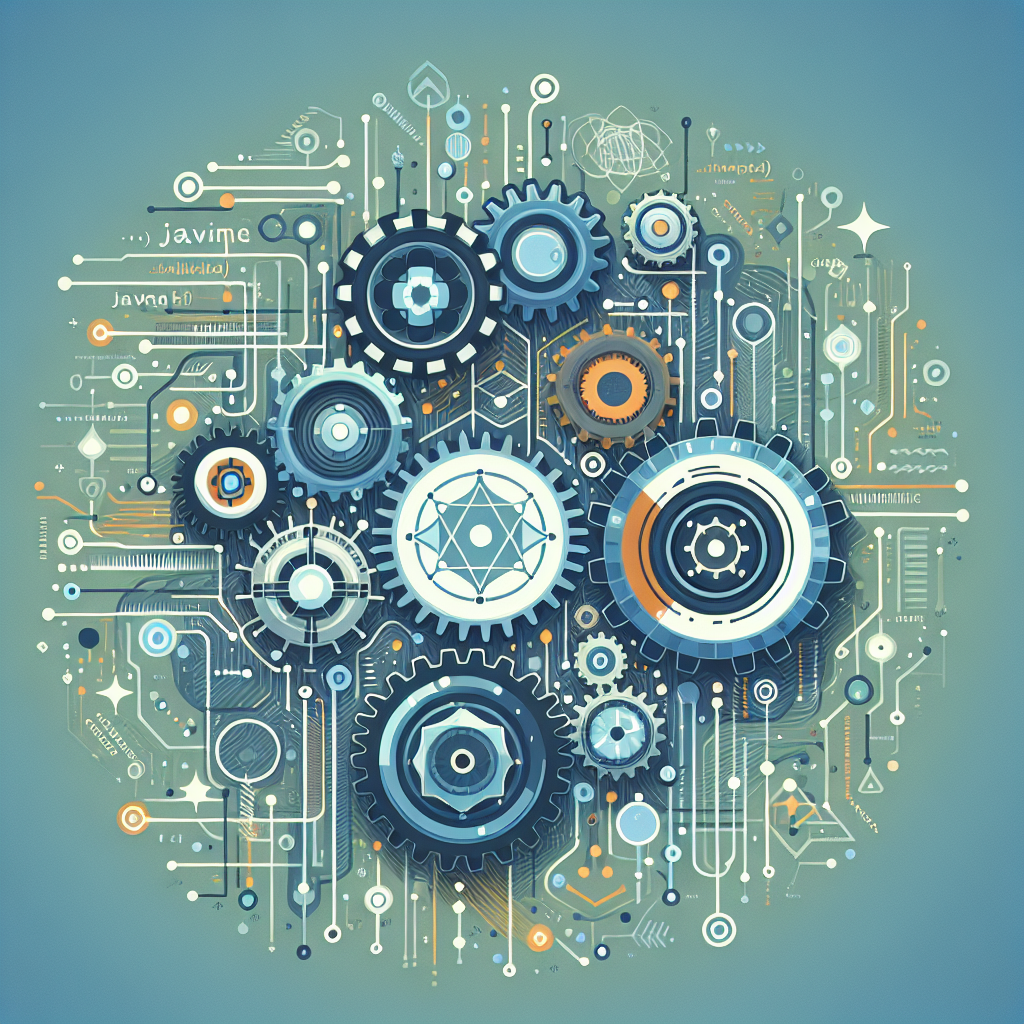
Understanding Synchronizers in Java
In the realm of Java concurrency, managing access to shared resources is crucial to ensure data consistency and avoid unexpected behavior. Synchronizers in Java offer a sophisticated mechanism to control synchronization among threads, ensuring that they work harmoniously without stepping on each other’s toes.
What Are Synchronizers?
Synchronizers are constructs that enable threads to coordinate their actions, allowing them to proceed only when certain conditions are met. They are designed to simplify complex multi-threading problems and provide a higher-level abstraction over traditional synchronization mechanisms like synchronized
blocks and methods.
Types of Synchronizers in Java
Java provides several built-in synchronizers in the java.util.concurrent
package. Each serves a unique purpose and is optimized for specific scenarios.
CountDownLatch
CountDownLatch is a synchronizer that allows one or more threads to wait until a set of operations being performed in other threads completes. It’s particularly useful in scenarios where a thread needs to wait for multiple threads to complete their tasks before proceeding.
import java.util.concurrent.CountDownLatch;
public class CountDownLatchExample {
private static final int TOTAL_THREADS = 3;
public static void main(String[] args) throws InterruptedException {
CountDownLatch latch = new CountDownLatch(TOTAL_THREADS);
for (int i = 0; i < TOTAL_THREADS; i++) {
new Thread(new Worker(latch)).start();
}
latch.await(); // Wait for all threads to finish
System.out.println("All threads have finished execution.");
}
static class Worker implements Runnable {
private final CountDownLatch latch;
Worker(CountDownLatch latch) {
this.latch = latch;
}
@Override
public void run() {
try {
// Simulate work
Thread.sleep(1000);
System.out.println(Thread.currentThread().getName() + " finished work.");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
latch.countDown();
}
}
}
}
CyclicBarrier
CyclicBarrier allows a set of threads to wait for each other to reach a common barrier point. It’s particularly useful when you have a fixed number of threads that need to repeatedly wait for each other.
import java.util.concurrent.BrokenBarrierException;
import java.util.concurrent.CyclicBarrier;
public class CyclicBarrierExample {
private static final int PARTIES = 3;
public static void main(String[] args) {
CyclicBarrier barrier = new CyclicBarrier(PARTIES, () -> System.out.println("All parties have arrived, let's proceed."));
for (int i = 0; i < PARTIES; i++) {
new Thread(new Task(barrier)).start();
}
}
static class Task implements Runnable {
private final CyclicBarrier barrier;
Task(CyclicBarrier barrier) {
this.barrier = barrier;
}
@Override
public void run() {
try {
System.out.println(Thread.currentThread().getName() + " is waiting at the barrier.");
barrier.await();
System.out.println(Thread.currentThread().getName() + " has crossed the barrier.");
} catch (InterruptedException | BrokenBarrierException e) {
Thread.currentThread().interrupt();
}
}
}
}
Semaphore
Semaphore is a more generalized form of synchronization which restricts the number of threads that can access a resource. It’s useful when you have a limited number of resources and you need to control access to them.
import java.util.concurrent.Semaphore;
public class SemaphoreExample {
private static final int PERMITS = 2;
public static void main(String[] args) {
Semaphore semaphore = new Semaphore(PERMITS);
for (int i = 0; i < 5; i++) {
new Thread(new ResourceUser(semaphore)).start();
}
}
static class ResourceUser implements Runnable {
private final Semaphore semaphore;
ResourceUser(Semaphore semaphore) {
this.semaphore = semaphore;
}
@Override
public void run() {
try {
semaphore.acquire();
System.out.println(Thread.currentThread().getName() + " acquired a permit.");
// Simulate resource usage
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
System.out.println(Thread.currentThread().getName() + " released a permit.");
semaphore.release();
}
}
}
}
Advantages of Using Synchronizers
Synchronizers provide several benefits when used correctly:
- Simplified Thread Coordination: They abstract the complexities involved in managing thread lifecycles and dependencies.
- Improved Resource Management: By restricting access to resources, synchronizers help prevent resource exhaustion.
- Enhanced Scalability: Synchronizers are designed to handle multiple threads efficiently, making them suitable for high-concurrency applications.
When to Use Synchronizers
Choosing the right synchronizer depends on the specific requirements of your application:
- Use CountDownLatch when you need a set number of threads to complete before proceeding.
- Opt for CyclicBarrier when you need threads to wait for each other repeatedly.
- Select Semaphore when limiting access to a set number of resources is necessary.
Conclusion
Synchronizers in Java are powerful tools for managing thread synchronization and coordination. By understanding their specific use cases and advantages, developers can effectively harness their potential to build robust, concurrent applications. As modern applications increasingly rely on multi-threading, mastering synchronizers becomes an essential skill for Java developers aiming to create efficient and scalable software.