Python Create Empty List: A Quick & Easy Guide
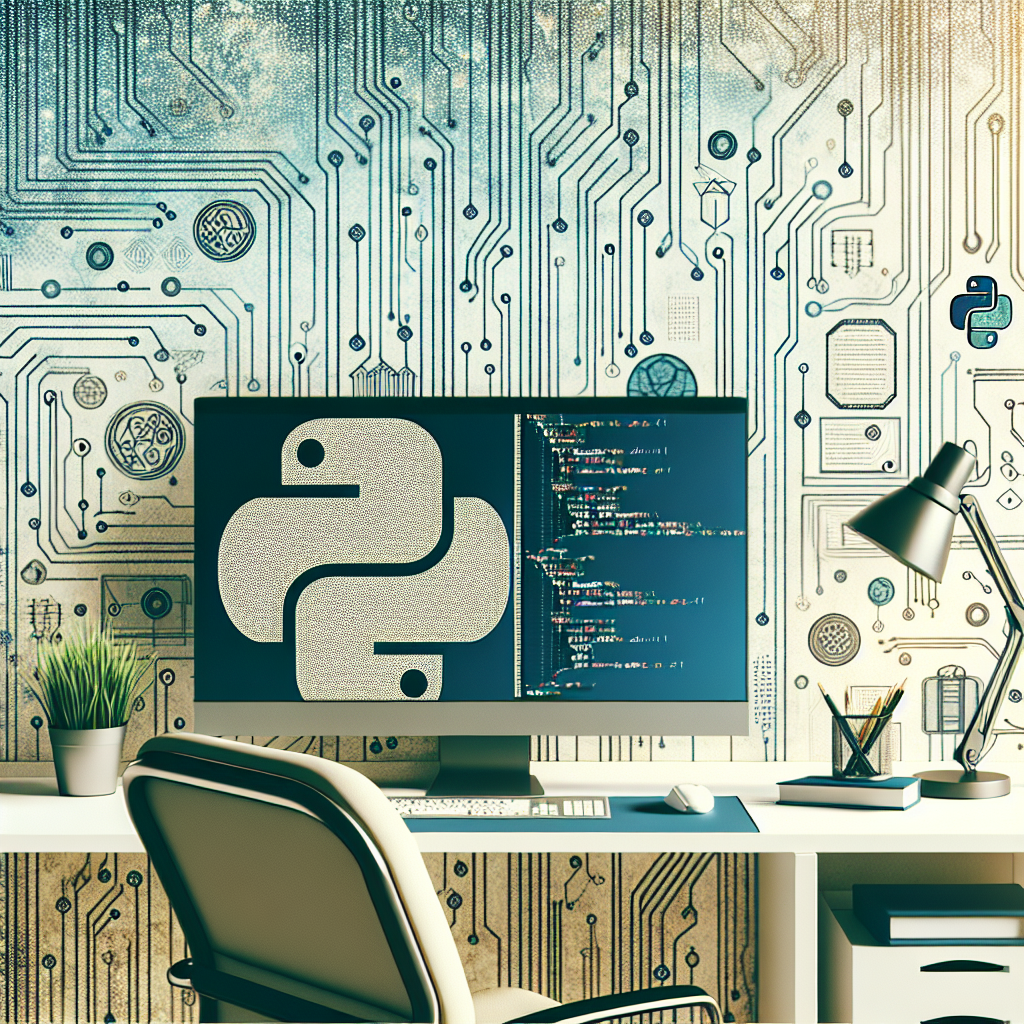
Introduction
Python is renowned for its simplicity and versatility, making it a favorite among both novice and seasoned developers. One of the fundamental aspects of Python programming involves working with lists. In this blog post, we’ll delve into the concept of creating an empty list in Python, a basic yet essential skill. We’ll also explore Python list initialization, various Python list methods, and Python list basics to provide a comprehensive understanding.
Python List Basics
Before diving into creating an empty list, let’s briefly review what a Python list is. A list in Python is a collection of items that are ordered and changeable. Lists are written with square brackets, and they allow duplicate members.
Characteristics of Python Lists
- Ordered: Lists maintain the order of items as they are added.
- Changeable: You can modify, add, or remove items after the list’s creation.
- Allow Duplicates: Lists can have items with the same value.
Python Create Empty List
Creating an empty list is a straightforward task in Python, and it’s often the first step in list manipulation.
How to Create an Empty List
There are primarily two ways to create an empty list in Python:
-
Using Square Brackets:
empty_list = []
-
Using the
list()
Constructor:empty_list = list()
Both methods will create an empty list, and neither is inherently better than the other. The choice between them depends on personal or project-specific coding style preferences.
Practical Example
Creating an empty list is often the precursor to appending data. Here’s an example of appending elements to an empty list:
fruits = [] # Initialize an empty list
fruits.append("apple")
fruits.append("banana")
In this example, we start with an empty list and use the append()
method to add items.
Python List Initialization
Initialization of lists is crucial when you want to predefine a list with a certain number or type of elements.
Predefined List with Values
You can initialize a list with predefined values:
numbers = [1, 2, 3, 4, 5]
List Comprehension
Python provides a powerful way to initialize lists using list comprehensions. This is particularly useful when you want to generate a list based on an existing sequence or range.
squares = [x**2 for x in range(10)]
This example creates a list of square numbers from 0 to 9.
Python List Methods
Python lists come with a variety of built-in methods that enhance their functionality. Understanding these methods is key to effective list manipulation.
Common List Methods
-
append()
: Adds an element to the end of the list.my_list.append("new item")
-
extend()
: Adds all elements from another iterable to the end of the list.my_list.extend(["item1", "item2"])
-
insert()
: Inserts an element at a specified position.my_list.insert(1, "inserted item")
-
remove()
: Removes the first occurrence of a specified value.my_list.remove("item")
-
pop()
: Removes and returns the element at the specified position.item = my_list.pop(2)
-
clear()
: Removes all elements from the list.my_list.clear()
Example of Using List Methods
Here’s an example showcasing the use of these methods:
animals = ["cat", "dog", "bird"]
animals.append("fish")
animals.insert(1, "hamster")
animals.remove("bird")
print(animals) # Output: ['cat', 'hamster', 'dog', 'fish']
This demonstrates the flexibility and power of Python list methods in managing data.
Conclusion
Understanding how to create and manipulate lists is a fundamental skill in Python programming. Whether you’re initializing an empty list for future use or using comprehensive list methods to manage data, mastering these basics is essential. Lists provide a dynamic way to handle collections of items, making them a vital tool in any Python developer’s arsenal. By leveraging the power of Python list initialization and methods, you can write more efficient and effective code.