Fix 'Access-Control-Allow-Origin' Errors: A Complete Guide
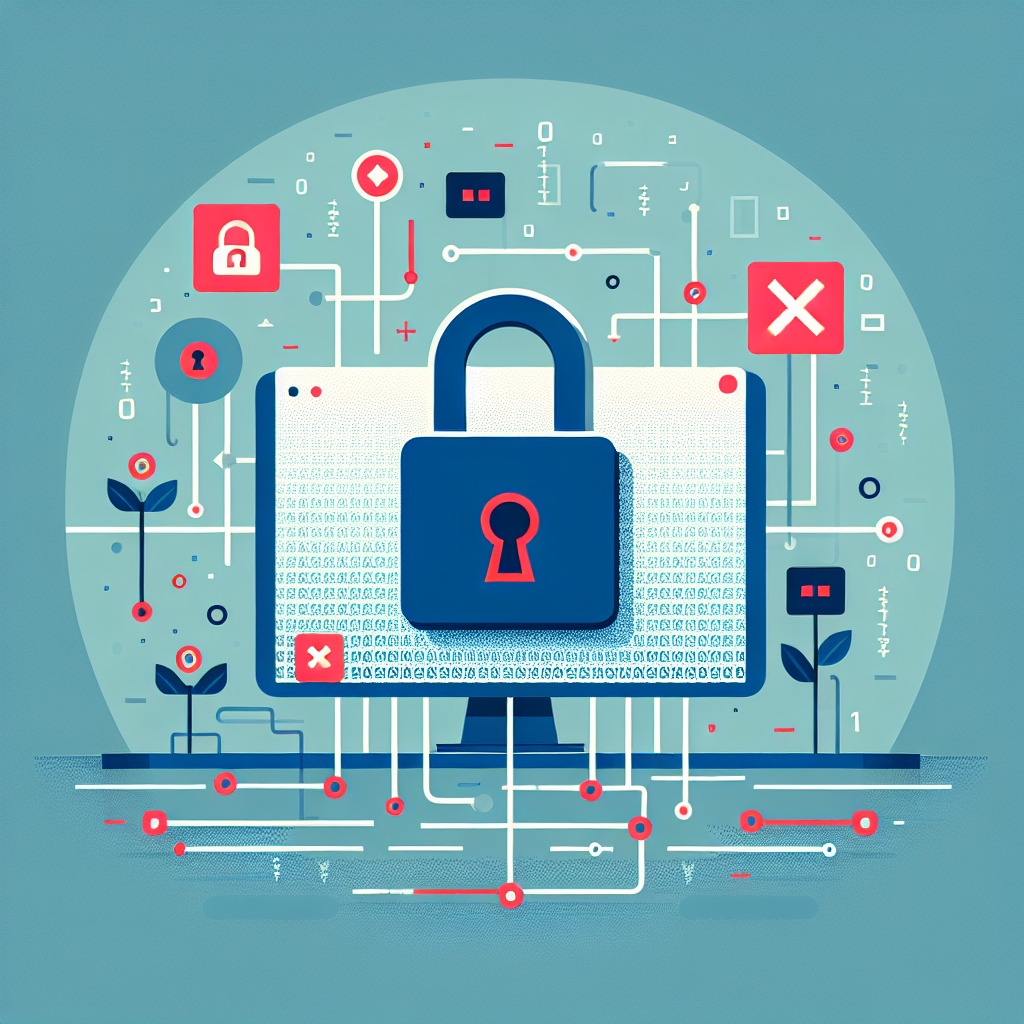
Understanding the ‘Access-Control-Allow-Origin’ Error
Web developers frequently encounter the “No ‘Access-Control-Allow-Origin’ header is present on the requested resource” error. This common issue arises when a web application attempts to access resources from a different domain, leading to a block by the browser due to security protocols. This article will delve into the specifics of this error, why it occurs, and how to resolve it.
What is Cross-Origin Resource Sharing (CORS)?
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers. It allows web applications from one origin to request resources from another origin, thereby enabling cross-origin requests. CORS is essential for maintaining security and preventing malicious activities, such as cross-site request forgery (CSRF).
Why the CORS Error Occurs
When a web application makes a cross-origin HTTP request, the browser checks the response for an Access-Control-Allow-Origin
header. If this header is missing, the browser blocks the request, resulting in the CORS error. Here are some common scenarios where this error might occur:
- API Calls from Different Domains: Accessing an API hosted on a different domain without the correct CORS configuration.
- Subdomain Issues: Attempting to access resources from a subdomain without proper headers.
- Local Development: Running a local server that makes requests to an external API without CORS setup.
How to Resolve the ‘Access-Control-Allow-Origin’ Error
Resolving the CORS error involves configuring the server to include the correct HTTP headers. Here are some strategies to address this issue:
1. Configuring the Server
Ensure your server includes the Access-Control-Allow-Origin
header in its responses. Here’s how you can set it up for different server environments:
-
Apache: Add the following lines to your
.htaccess
file:<IfModule mod_headers.c> Header set Access-Control-Allow-Origin "*" </IfModule>
-
Nginx: Add this configuration to the server block:
add_header 'Access-Control-Allow-Origin' '*';
-
Node.js (Express): Use middleware to set the header:
app.use((req, res, next) => { res.header("Access-Control-Allow-Origin", "*"); next(); });
2. Allow Specific Domains
For enhanced security, it’s advisable to allow specific domains instead of using a wildcard (*
). Replace the wildcard with the origin you want to allow:
-
Example for Express:
app.use((req, res, next) => { res.header("Access-Control-Allow-Origin", "https://example.com"); next(); });
3. Handling Preflight Requests
Browsers send a preflight request with the HTTP method OPTIONS to verify whether the actual request is safe. Ensure your server can handle these requests:
-
Express Example:
app.options('*', (req, res) => { res.header("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE"); res.send(); });
Best Practices for Managing CORS
To manage CORS effectively, consider these best practices:
- Use Middleware: Implement middleware to handle CORS headers dynamically, especially if you’re working with Node.js and Express.
- Whitelist Trusted Domains: Instead of allowing all domains, maintain a list of trusted domains to minimize security risks.
- Test in Different Environments: Ensure your CORS configuration works across different development, staging, and production environments.
Common Misconceptions About CORS
While CORS is crucial for security, misunderstandings can lead to improper configurations:
- CORS is Not a Server-Side Protection: It’s a browser feature designed to protect users, not a mechanism to secure server data.
- The Wildcard Can Be Dangerous: Allowing all origins with
*
is risky, especially for sensitive applications. Always prefer a whitelist approach.
Conclusion
The “No ‘Access-Control-Allow-Origin’ header is present on the requested resource” error can be frustrating, but understanding CORS and properly configuring your server can resolve it. Implementing the right headers, handling preflight requests, and following best practices ensure your web applications communicate securely across domains. By addressing CORS errors, developers can improve both the security and functionality of their web applications.