Master Mockito: Effortlessly Mock Static Methods
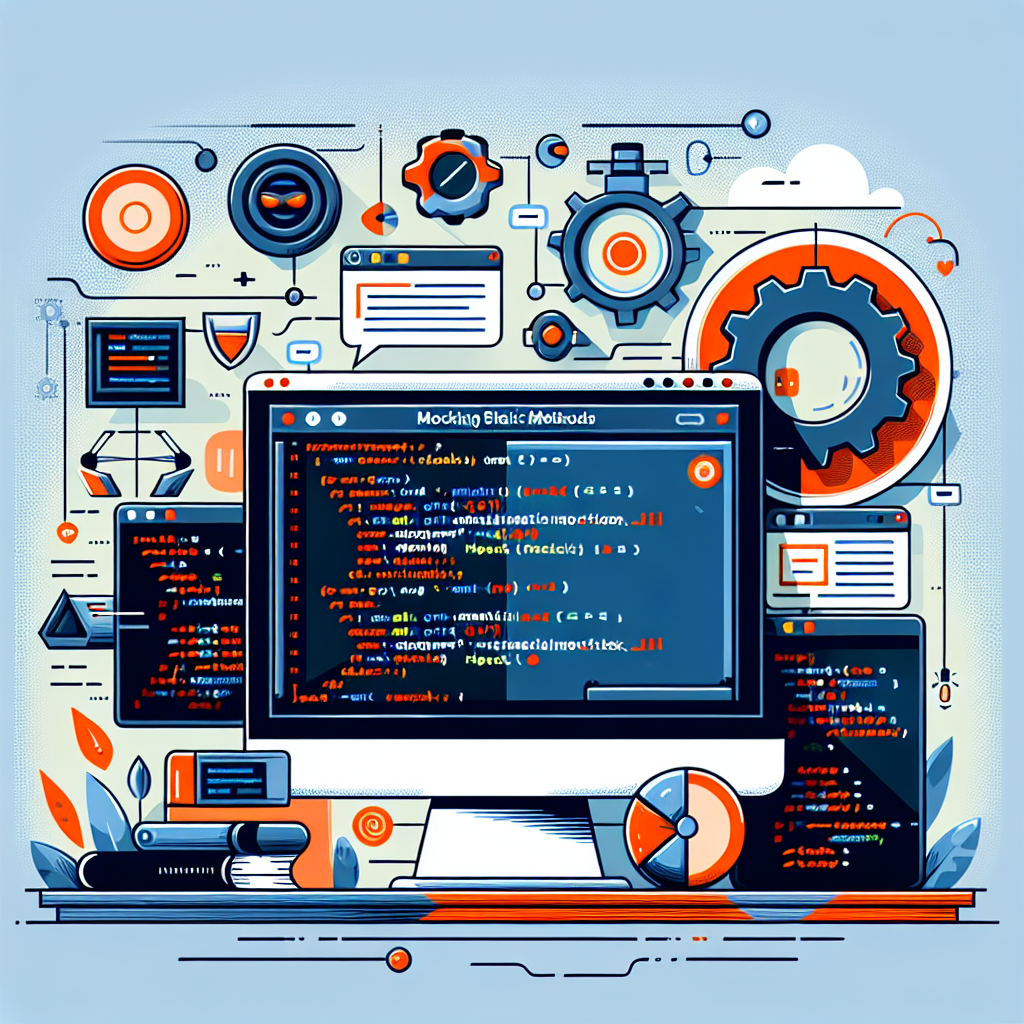
Understanding How to Mock Static Methods with Mockito
In the evolving landscape of software development, unit testing stands as a cornerstone for ensuring code reliability. However, developers often encounter challenges when dealing with static methods. Traditionally, static methods have been notoriously difficult to mock due to their fixed behavior. Enter Mockito—a powerful mocking framework that simplifies this task. This post delves into the intricacies of mocking static methods using Mockito, providing you with a comprehensive guide to enhance your unit testing process.
Why Mock Static Methods?
Static methods are frequently used in utility classes and can offer significant performance benefits. However, their rigid nature poses challenges in testing. Mocking static methods is essential for:
- Isolating Tests: Allows testing of a single unit without external dependencies.
- Controlling Behavior: Provides the ability to simulate various scenarios and edge cases.
- Improving Test Coverage: Ensures every path in the static method is tested.
Incorporating these practices can lead to robust, maintainable codebases.
Mockito Tutorial: Setting Up Your Environment
Before diving into static method mocking, ensure your development environment is ready. Follow these steps to set up Mockito:
-
Add Dependencies: Include Mockito in your project’s build file.
- For Maven:
<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-core</artifactId> <version>4.0.0</version> <scope>test</scope> </dependency>
- For Gradle:
testImplementation 'org.mockito:mockito-core:4.0.0'
- For Maven:
-
Enable Inline Mocking: Mockito requires an additional library to mock static methods.
- For Maven:
<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-inline</artifactId> <version>4.0.0</version> <scope>test</scope> </dependency>
- For Gradle:
testImplementation 'org.mockito:mockito-inline:4.0.0'
- For Maven:
Static Method Mocking with Mockito
Now that your environment is configured, let’s explore how to mock static methods using Mockito.
Writing a Simple Static Method
Consider a utility class with a static method:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
Mocking the Static Method
To mock the add
method, follow these steps:
-
Use the
mockStatic
Method: Initiate a mock for the static method.import static org.mockito.Mockito.*; import org.junit.jupiter.api.Test; import org.mockito.MockedStatic; public class MathUtilsTest { @Test void testAdd() { try (MockedStatic<MathUtils> utilities = mockStatic(MathUtils.class)) { utilities.when(() -> MathUtils.add(1, 2)).thenReturn(5); int result = MathUtils.add(1, 2); assertEquals(5, result); } } }
- Open a Mocked Context: Use a try-with-resources block for
MockedStatic
. - Specify Behavior: Use
when().thenReturn()
for defining the behavior ofMathUtils.add()
.
- Open a Mocked Context: Use a try-with-resources block for
Benefits of Static Method Mocking
- Enhanced Flexibility: Easily test different return values and scenarios.
- Improved Test Isolation: Focus on testing the unit without interference from static methods.
- Reduced Code Complexity: Simplifies testing of complex static behaviors.
Best Practices for Unit Testing with Mockito
When using Mockito for static method mocking, adhere to these best practices:
- Limit Mocking: Only mock static methods when necessary to avoid over-reliance on mocks.
- Maintain Readability: Keep tests clean and easy to understand.
- Use Proper Assertions: Ensure that assertions accurately reflect the expected outcomes.
- Embrace Test-Driven Development (TDD): Write tests before implementing functionality for more reliable code.
Conclusion: Elevate Your Testing with Mockito
Mastering the art of mocking static methods with Mockito empowers developers to create more reliable and maintainable software. By isolating tests and controlling static method behavior, you can achieve comprehensive test coverage and robust application logic. Remember, while Mockito is a powerful tool, it should be used judiciously to maintain clarity and efficiency in your tests. Integrate these practices into your development workflow and watch your code quality soar.
Incorporate these insights into your projects to harness the full potential of Mockito and elevate your unit testing strategy.