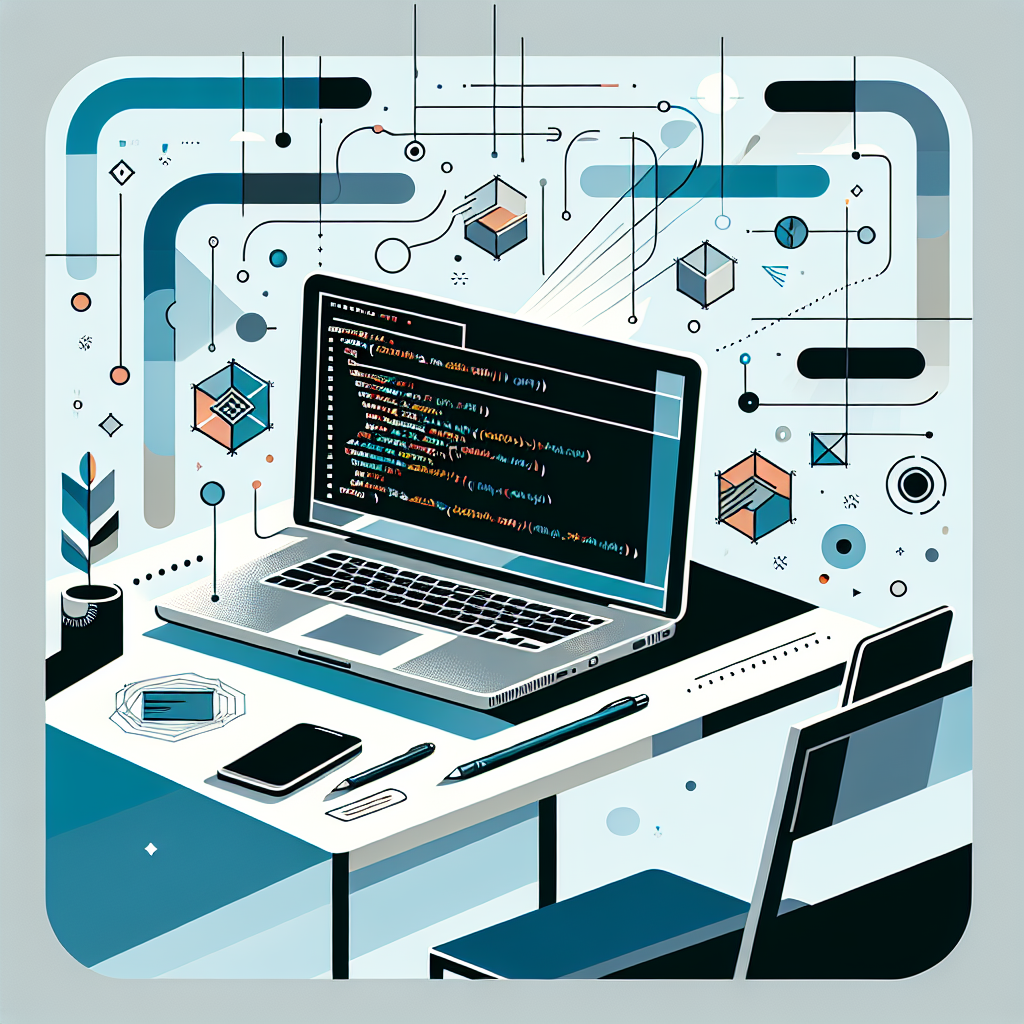
Understanding JSON Multiline Strings
JSON, or JavaScript Object Notation, is a lightweight data interchange format that’s easy for humans to read and write. It’s also easy for machines to parse and generate. One of the challenges developers often face when working with JSON is handling multiline strings. This blog post will explore how JSON handles multiline strings, provide practical examples, and offer solutions to common issues.
What Are JSON Multiline Strings?
In JSON, strings are typically written on a single line. However, there are scenarios where you might need to include a string that spans multiple lines, such as when dealing with lengthy text or formatted content. This poses a challenge because JSON does not natively support multiline strings like some other programming languages.
How JSON Handles Strings
JSON strings are always enclosed in double quotes. Characters such as line breaks and tabs are represented using escape sequences. For instance:
- Line break:
\n
- Tab:
\t
- Double quote:
\"
Incorporating Multiline Strings in JSON
To include multiline strings in JSON, you must use escape sequences. Here’s how you can do it:
{
"description": "This is a multiline string.\nIt requires the use of escape sequences\nto maintain formatting."
}
Each line of the string is separated by \n
, allowing it to appear as a multiline string when parsed by JSON-compatible systems.
Common Use Cases for JSON Multiline Strings
- Storing Large Text Blocks: When dealing with large bodies of text, such as descriptions or documentation, multiline strings can help maintain readability.
- Configuration Files: Multiline strings can be used in configuration files to store code snippets or SQL queries.
- Internationalization: Multiline strings can handle text in different languages, where text formatting varies.
Challenges with JSON Multiline Strings
- Readability: Writing JSON multiline strings with escape sequences can reduce readability.
- Error-Prone: Manually inserting escape sequences can lead to errors if not done carefully.
- Limited Tool Support: Some tools and editors may not render escape sequences correctly, leading to confusion.
Solutions and Best Practices
Use String Concatenation
In some programming environments, you can concatenate strings to create multiline strings before serializing them into JSON:
const multilineString = "This is a multiline string.\n" +
"It is created using string concatenation.";
const jsonString = JSON.stringify({ description: multilineString });
Leverage Template Literals
In JavaScript, template literals make it easier to handle multiline strings. Though JSON itself doesn’t support them, they can be used to prepare strings before converting them to JSON:
const multilineString = `This is a multiline string.
It is created using template literals.`;
const jsonString = JSON.stringify({ description: multilineString });
Use External Libraries
Libraries like YAML or specialized JSON serializers can help manage multiline strings more elegantly:
const yaml = require('js-yaml');
const obj = yaml.load(`
description: |
This is a multiline string.
It is loaded using a YAML parser.
`);
JSON Multiline Strings in Different Programming Languages
Python
In Python, you can use triple quotes to define multiline strings, which can then be incorporated into JSON:
import json
multiline_string = """This is a multiline string.
It spans multiple lines."""
json_data = json.dumps({"description": multiline_string})
Java
Java’s String
class includes methods to handle multiline strings that can be serialized into JSON:
import org.json.JSONObject;
String multilineString = "This is a multiline string.\nIt spans multiple lines.";
JSONObject json = new JSONObject();
json.put("description", multilineString);
Conclusion
Handling multiline strings in JSON requires careful attention to detail, particularly with the use of escape sequences. By understanding the challenges and solutions, developers can effectively manage multiline strings in their JSON data. Whether you’re using string concatenation, template literals, or external libraries, choosing the right approach can ensure your JSON remains both human-readable and machine-friendly. As JSON continues to be a cornerstone in data interchange, mastering these techniques is essential for any modern developer.