Master Java Comparator: Boost Sorting Efficiency Now
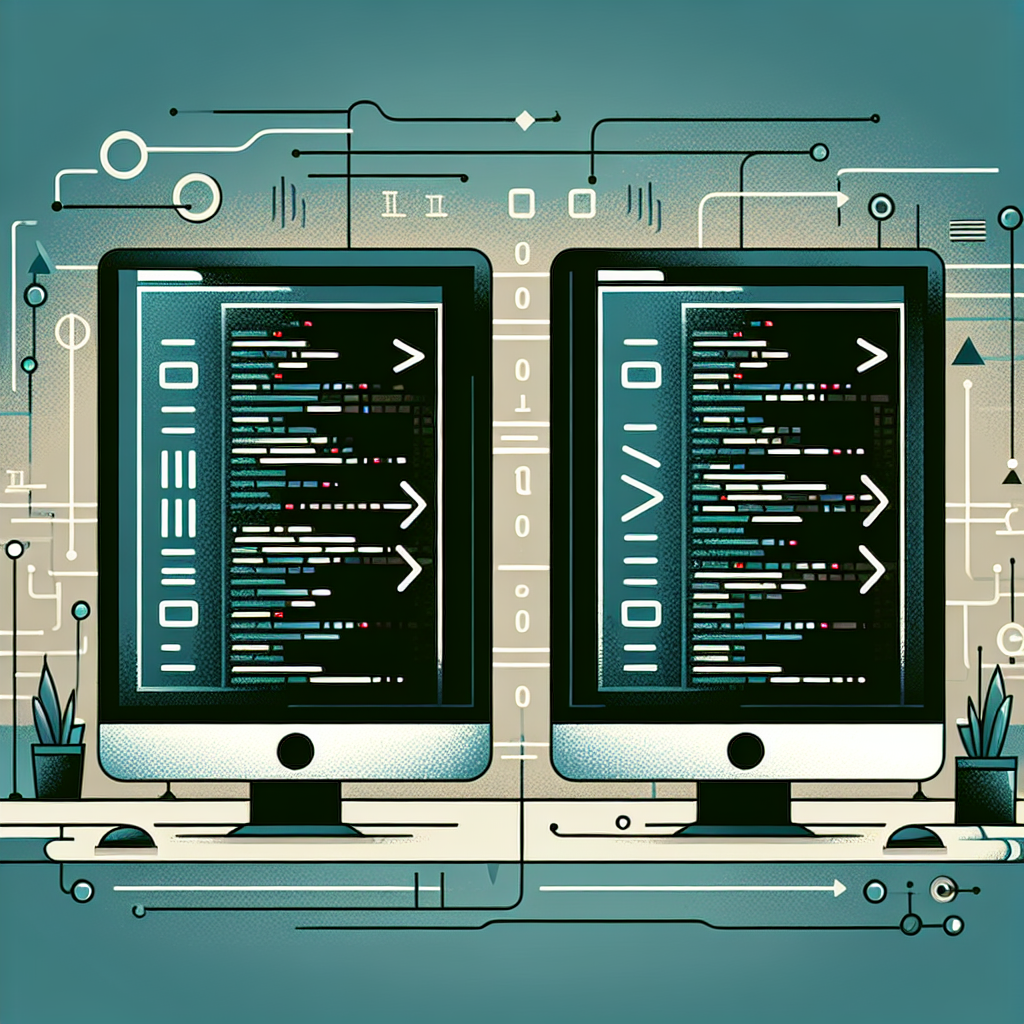
Introduction
When it comes to sorting collections in Java, the Comparator
interface plays a pivotal role. It allows developers to define custom sorting logic, making it an essential tool in Java sorting techniques. Whether you’re sorting user-defined objects or need a specific order, understanding Java’s Comparator
is crucial. This Java Comparator Guide will delve into how to use comparators, how they differ from Comparable
, and how you can implement custom comparators to suit your needs.
Understanding Java Comparator
The Java Comparator
is a functional interface in the java.util
package. It allows for ordering collections using a custom comparator logic. This interface is particularly useful when the natural ordering of objects, defined by the Comparable
interface, is not sufficient.
Key Features of Comparator
- Custom Sorting: Define custom sorting logic for collections.
- Functional Interface: Implement using lambda expressions since Java 8.
- Multiple Sorting: Sort objects on multiple fields.
Comparator vs Comparable
While both Comparator
and Comparable
are used for sorting, they serve different purposes. Comparable
is used for natural ordering, typically implemented within the class itself. In contrast, Comparator
is used for custom ordering and can be implemented externally.
- Comparable: Implemented in the object class.
- Comparator: Implemented as a separate class or lambda.
Implementing a Custom Comparator
Implementing a custom comparator involves overriding the compare
method. This method takes two arguments and returns an integer indicating their relative order.
Basic Comparator Example
Below is a simple example of using a custom comparator to sort a list of integers in descending order:
import java.util.*;
public class ComparatorExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(5, 3, 8, 1, 4);
Comparator<Integer> descendingOrder = new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2.compareTo(o1);
}
};
Collections.sort(numbers, descendingOrder);
System.out.println(numbers); // Output: [8, 5, 4, 3, 1]
}
}
Using Lambda Expressions
With the advent of Java 8, lambda expressions have simplified the way we implement comparators.
List<Integer> numbers = Arrays.asList(5, 3, 8, 1, 4);
numbers.sort((o1, o2) -> o2.compareTo(o1));
System.out.println(numbers); // Output: [8, 5, 4, 3, 1]
Using lambdas makes the code more concise and readable, which is ideal for modern Java development.
Advanced Java Sorting Techniques
Beyond simple sorting, Java comparators can handle more complex sorting scenarios. This includes multi-level sorting and sorting based on multiple criteria.
Sorting with Multiple Criteria
Suppose you have a list of Person
objects that you want to sort by age and then by name. Here’s how you can achieve this:
import java.util.*;
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return name + " : " + age;
}
}
public class MultiCriteriaSorting {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("Alice", 30),
new Person("Bob", 25),
new Person("Charlie", 30),
new Person("David", 20)
);
Comparator<Person> byAgeThenName = Comparator
.comparingInt((Person p) -> p.age)
.thenComparing(p -> p.name);
Collections.sort(people, byAgeThenName);
System.out.println(people);
// Output: [David : 20, Bob : 25, Alice : 30, Charlie : 30]
}
}
Using Comparator
with Streams
Java Streams provide a powerful way to process collections, and you can use comparators to sort elements within streams.
List<Person> sortedPeople = people.stream()
.sorted(Comparator.comparingInt((Person p) -> p.age)
.thenComparing(p -> p.name))
.collect(Collectors.toList());
System.out.println(sortedPeople);
Streams offer a fluent way to handle data transformation and sorting, making your code more expressive and functional.
Conclusion
Understanding and utilizing the Java Comparator interface is a vital skill for any Java developer. From basic sorting to advanced multi-criteria sorting, comparators provide the flexibility needed for various scenarios. By implementing custom comparators and leveraging Java’s functional programming features, you can efficiently manage complex sorting tasks. Remember, the right sorting technique can significantly optimize the performance of your application, ensuring efficient data handling and user satisfaction.