Master Python's Increment Operator: Boost Your Skills
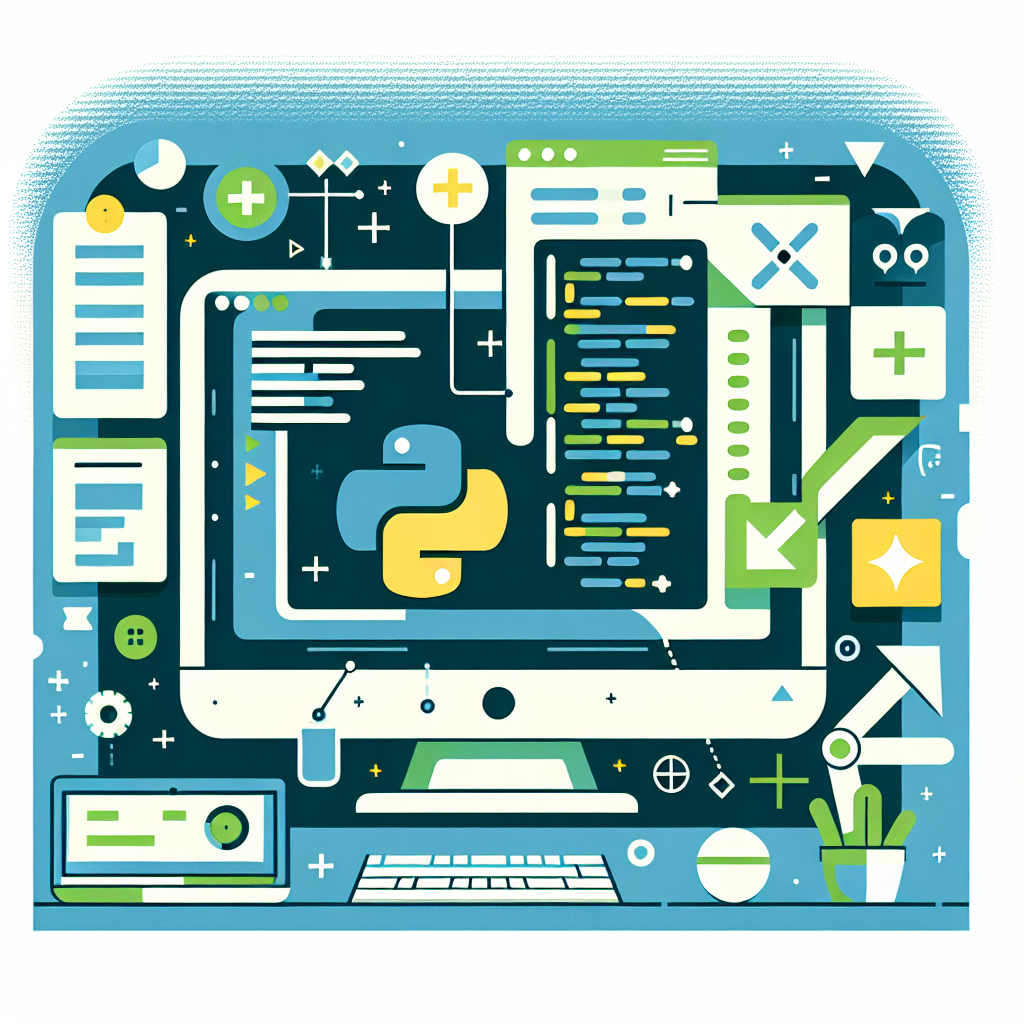
Understanding the Python Increment Operator
Python is a versatile and widely-used programming language known for its simplicity and readability. However, newcomers often find themselves puzzled by Python’s approach to incrementing variables. Unlike some other languages, Python does not have a dedicated increment operator like ++
. In this blog post, we will explore Python’s syntax for incrementing values, how to perform increments in Python, and best practices for updating variables.
Python Syntax and the Absence of ++
In languages like C++ or Java, the increment operator ++
is commonly used to increase the value of an integer by one. For example, in C++, you might see:
int a = 5;
a++; // a is now 6
However, Python does not support ++
or --
operators. This design choice aligns with Python’s philosophy of simplicity and explicitness. Instead, Python encourages the use of more explicit operations to achieve the same result.
How to Perform Increment in Python
To increment a variable in Python, you use the +=
operator. This operator combines addition and assignment in one step. Here is how it works:
## Initializing a variable
counter = 0
## Incrementing the variable
counter += 1
print(counter) # Output: 1
This code snippet illustrates how to update a Python variable by adding one to its current value. The +=
operator is straightforward and widely used in Python programming.
Why Python Prefers +=
Python’s preference for +=
over ++
is rooted in its aim for clarity and readability. The +=
operator is explicit about what it does: it adds a specified value to the variable. This explicitness reduces the potential for misunderstandings or errors, especially in complex codebases.
Other Ways to Increment Variables
While += 1
is the most common way to increment a value, Python offers other methods that can be useful in different contexts. Here are a few alternatives:
-
Standard Addition Assignment: This method uses the standard addition and assignment operators separately.
counter = 0 counter = counter + 1
-
Using Functions: You can encapsulate the increment operation within a function for reusability.
def increment(value): return value + 1 counter = 0 counter = increment(counter)
-
Using Loops: In loops, incrementing is often an integral part of the iteration process.
for i in range(5): print(i) # Outputs: 0, 1, 2, 3, 4
Practical Applications of Incrementing
Incrementing variables is a fundamental operation in programming. It’s used in various scenarios, such as:
- Counting: Keeping track of occurrences or iterations.
- Indexing: Navigating through lists or arrays.
- Loop Control: Managing the number of iterations in loops.
Consider a scenario where you need to count the number of even numbers in a list:
numbers = [1, 2, 3, 4, 5, 6]
even_count = 0
for number in numbers:
if number % 2 == 0:
even_count += 1
print(even_count) # Output: 3
This example demonstrates how the increment operation plays a crucial role in accumulating a count during a loop.
Best Practices for Incrementing in Python
When updating variables in Python, consider the following best practices:
- Use Descriptive Variable Names: Ensure variable names clearly indicate their purpose, enhancing code readability.
- Avoid Unnecessary Increments: Only increment variables when necessary for your application’s logic.
- Leverage Python’s Built-in Functions: For complex data manipulation, explore Python’s rich set of built-in functions and libraries.
Conclusion
While Python does not have a dedicated increment operator like ++
, it provides flexible and clear ways to perform increment operations using +=
and other methods. This approach aligns with Python’s philosophy of simplicity and readability, making it a preferred choice for developers worldwide. By understanding and utilizing Python’s syntax for incrementing, you can write efficient and maintainable code.
Incrementing is a fundamental concept in programming, and mastering it in Python will enhance your coding skills and improve your ability to implement effective solutions in a wide range of applications.