Golang Google Sheets Tutorial: Master Integration Fast
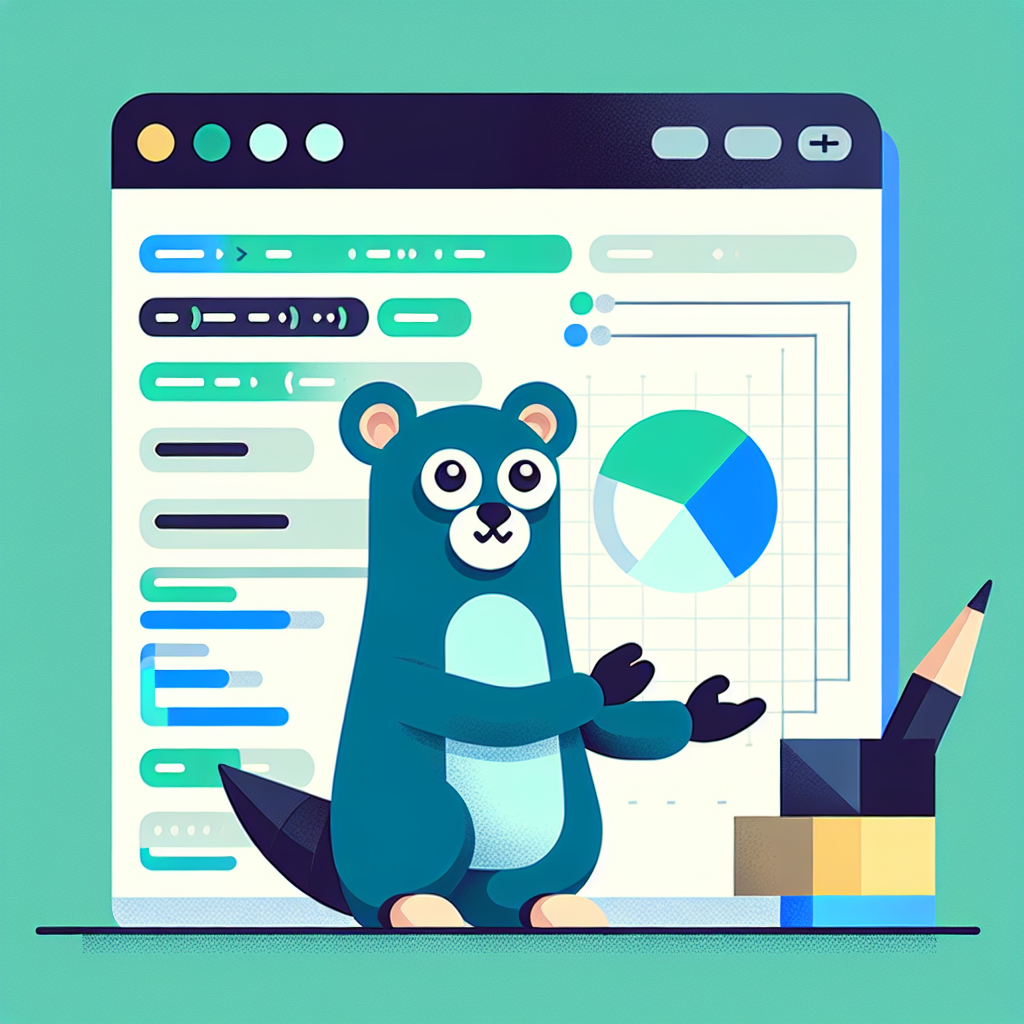
Introduction
In today’s digital age, the ability to automate tasks and manipulate data efficiently is paramount. With Google Sheets being one of the most widely used spreadsheet tools, integrating it with powerful programming languages like Golang offers a myriad of possibilities. This Golang Google Sheets tutorial will guide you through the process of using the Golang sheets API to automate tasks and manage data. Whether you are looking to perform data manipulation or automate repetitive tasks, this guide will provide the foundational knowledge you need.
Setting Up Your Environment
Before diving into code, you need to set up your environment. This involves installing Golang and configuring the Google Sheets API.
Installing Golang
- Download Golang: Visit the official Golang website and download the latest version for your operating system.
- Install Golang: Follow the installation instructions provided on the site. Ensure that Golang is installed correctly by running
go version
in your terminal.
Enabling Google Sheets API
- Create a Project: Go to the Google Cloud Console and create a new project.
- Enable the API: Navigate to the API & Services dashboard and enable the Google Sheets API.
- Set Up Credentials: Create credentials for the API. Choose OAuth 2.0 client IDs and download the JSON file. Save it securely as you will need it for authentication.
Connecting Golang to Google Sheets
With your environment ready, the next step is to establish a connection between Golang and Google Sheets.
Authenticating with the API
To interact with the Google Sheets API, you’ll need to authenticate using the credentials obtained earlier. Here’s how to set it up in Golang:
package main
import (
"context"
"encoding/json"
"fmt"
"io/ioutil"
"log"
"net/http"
"os"
"golang.org/x/oauth2/google"
"google.golang.org/api/sheets/v4"
)
// Reads credentials and returns a client
func getClient(config *oauth2.Config) *http.Client {
tokFile := "token.json"
tok, err := tokenFromFile(tokFile)
if err != nil {
tok = getTokenFromWeb(config)
saveToken(tokFile, tok)
}
return config.Client(context.Background(), tok)
}
// Other helper functions like tokenFromFile, getTokenFromWeb, and saveToken would be defined here...
Accessing a Google Sheet
Once authenticated, you can access and manipulate Google Sheets. Here is a simple example of how to read data from a Google Sheet:
func main() {
ctx := context.Background()
b, err := ioutil.ReadFile("credentials.json")
if err != nil {
log.Fatalf("Unable to read client secret file: %v", err)
}
// If modifying these scopes, delete your previously saved token.json.
config, err := google.ConfigFromJSON(b, sheets.SpreadsheetsReadonlyScope)
if err != nil {
log.Fatalf("Unable to parse client secret file to config: %v", err)
}
client := getClient(config)
srv, err := sheets.New(client)
if err != nil {
log.Fatalf("Unable to retrieve Sheets client: %v", err)
}
spreadsheetId := "your-spreadsheet-id"
readRange := "Sheet1!A1:D10"
resp, err := srv.Spreadsheets.Values.Get(spreadsheetId, readRange).Do()
if err != nil {
log.Fatalf("Unable to retrieve data from sheet: %v", err)
}
if len(resp.Values) == 0 {
fmt.Println("No data found.")
} else {
fmt.Println("Name, Major:")
for _, row := range resp.Values {
// Print columns A and B, which correspond to indices 0 and 1.
fmt.Printf("%s, %s\n", row[0], row[1])
}
}
}
Manipulating Data with Golang
Once you have successfully accessed your Google Sheets data, the next step is data manipulation. This process involves reading, updating, or deleting data efficiently.
Reading Data
Reading data involves fetching specific cell values or ranges. Use the Values.Get()
method as shown in the example above to fetch the required data.
Writing Data
To update or append data in your Google Sheet, use the Values.Update()
or Values.Append()
methods. Here’s an example of how to append data:
writeRange := "Sheet1!A11"
valueInputOption := "RAW"
valueRange := &sheets.ValueRange{
Values: [][]interface{}{
{"New Name", "New Major"},
},
}
_, err = srv.Spreadsheets.Values.Append(spreadsheetId, writeRange, valueRange).ValueInputOption(valueInputOption).Do()
if err != nil {
log.Fatalf("Unable to append data: %v", err)
}
Automating Google Sheets with Golang
Automation is a significant advantage of using Golang with Google Sheets. Automate routine tasks like report generation, data consolidation, and more.
Scheduling Tasks
Use Golang’s time
package to schedule tasks. You can set intervals for your program to run specific functions, automating your workflow seamlessly.
package main
import (
"fmt"
"time"
)
func main() {
ticker := time.NewTicker(24 * time.Hour)
for {
select {
case <-ticker.C:
fmt.Println("Running scheduled task...")
// Call your function to automate a task
}
}
}
Conclusion
Integrating Golang with Google Sheets opens up a world of possibilities for Google Sheets automation and data manipulation. By leveraging the power of the Golang sheets API, you can streamline workflows, enhance productivity, and focus on more strategic tasks. As you delve deeper into this integration, you’ll discover even more ways to optimize your processes, making this powerful combination an invaluable tool in your software development toolkit.