Master Golang Google Analytics Setup in Minutes
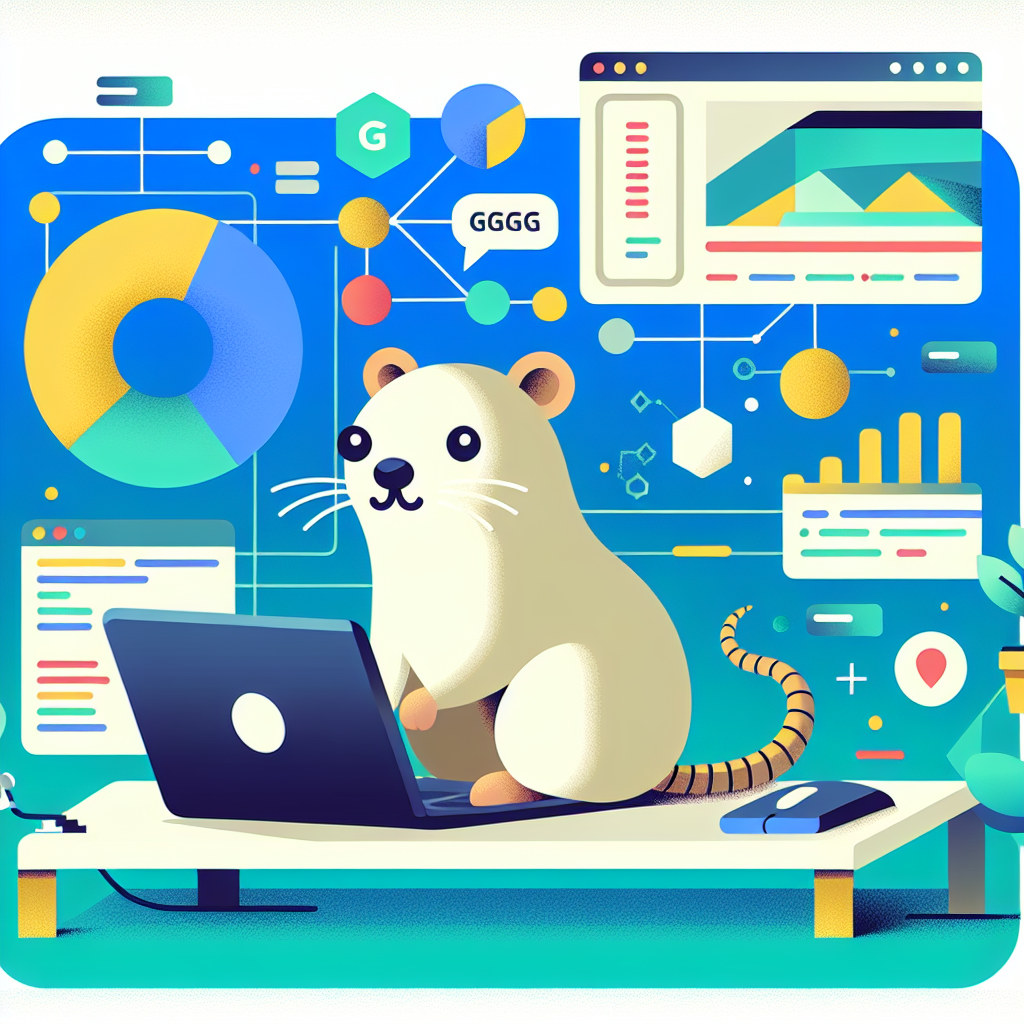
Introduction
In today’s digital age, understanding user interactions on your website is crucial for improving user experience and optimizing marketing strategies. Google Analytics is a powerful tool used by millions of developers to gain insights into web traffic and user behavior. If you’re a Go developer, integrating Google Analytics into your Golang application is a practical way to monitor your web analytics. This guide will walk you through a step-by-step process to set up Golang Google Analytics, ensuring you capture essential tracking data efficiently.
Why Use Golang with Google Analytics?
Golang, known for its performance and simplicity, is increasingly popular for building web applications. Integrating Google Analytics with Golang allows developers to leverage the language’s speed and efficiency while collecting valuable analytics data. This setup helps you understand user behavior, track conversion rates, and improve overall site performance.
Setting Up Google Analytics
Creating a Google Analytics Account
Before integrating Google Analytics with Golang, you need to create an account:
- Sign Up: Visit the Google Analytics website and sign up.
- Create a Property: Set up a new property for your website or application to generate a tracking ID.
- Get the Tracking ID: After creating a property, you will receive a tracking ID (e.g., UA-XXXXXXXXX-X).
Configuring Google Analytics
Once you have your tracking ID, configure Google Analytics for your Golang application.
- JavaScript Snippet: For web-based applications, insert the JavaScript snippet provided by Google Analytics into your HTML files. This snippet is essential for initial tracking.
<script async src="https://www.googletagmanager.com/gtag/js?id=UA-XXXXXXXXX-X"></script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'UA-XXXXXXXXX-X');
</script>
Integrating Google Analytics with Golang
Setting Up a Basic Golang Web Server
To track events and page visits, set up a basic Golang web server. Here’s a simple example using the net/http
package:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome to my Golang web application!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
Sending Data to Google Analytics
To send custom data to Google Analytics, utilize HTTP requests. Here’s how to track a page view:
package main
import (
"net/http"
"net/url"
"log"
)
func trackPageView(clientID, trackingID, documentPath string) {
endpoint := "https://www.google-analytics.com/collect"
data := url.Values{
"v": {"1"},
"tid": {trackingID},
"cid": {clientID},
"t": {"pageview"},
"dp": {documentPath},
}
resp, err := http.PostForm(endpoint, data)
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
}
func main() {
clientID := "555" // Random unique client ID
trackingID := "UA-XXXXXXXXX-X" // Replace with your tracking ID
documentPath := "/home"
trackPageView(clientID, trackingID, documentPath)
}
Using Third-Party Libraries
To simplify the integration, consider using a third-party library like go-analytics
. These libraries provide higher-level abstractions for sending data to Google Analytics:
- Install the Library: Use Go modules to add the dependency.
go get github.com/example/go-analytics
- Send Data: Utilize the library’s functions to send tracking data.
package main
import (
"github.com/example/go-analytics"
)
func main() {
analytics := analytics.New("UA-XXXXXXXXX-X")
analytics.PageView("555", "/home")
}
Best Practices for Golang Google Analytics Integration
Ensure Data Accuracy
- Use unique client IDs to distinguish users.
- Validate data before sending to avoid incorrect tracking.
Optimize Performance
- Batch requests to minimize network overhead.
- Use asynchronous requests to prevent blocking operations.
Monitor and Analyze Data
- Regularly review analytics reports.
- Set up custom dashboards for specific metrics.
Conclusion
Integrating Google Analytics with your Golang application is a strategic move for understanding user behavior and enhancing web analytics. By setting up tracking through Golang, you gain precise insights into user interactions and can make informed decisions to improve your application. Follow these steps and best practices to ensure a smooth and effective Golang Google Analytics setup. With the right implementation, you can leverage the full potential of web analytics to drive growth and success.