Golang Concurrency: Master Parallel Programming Today
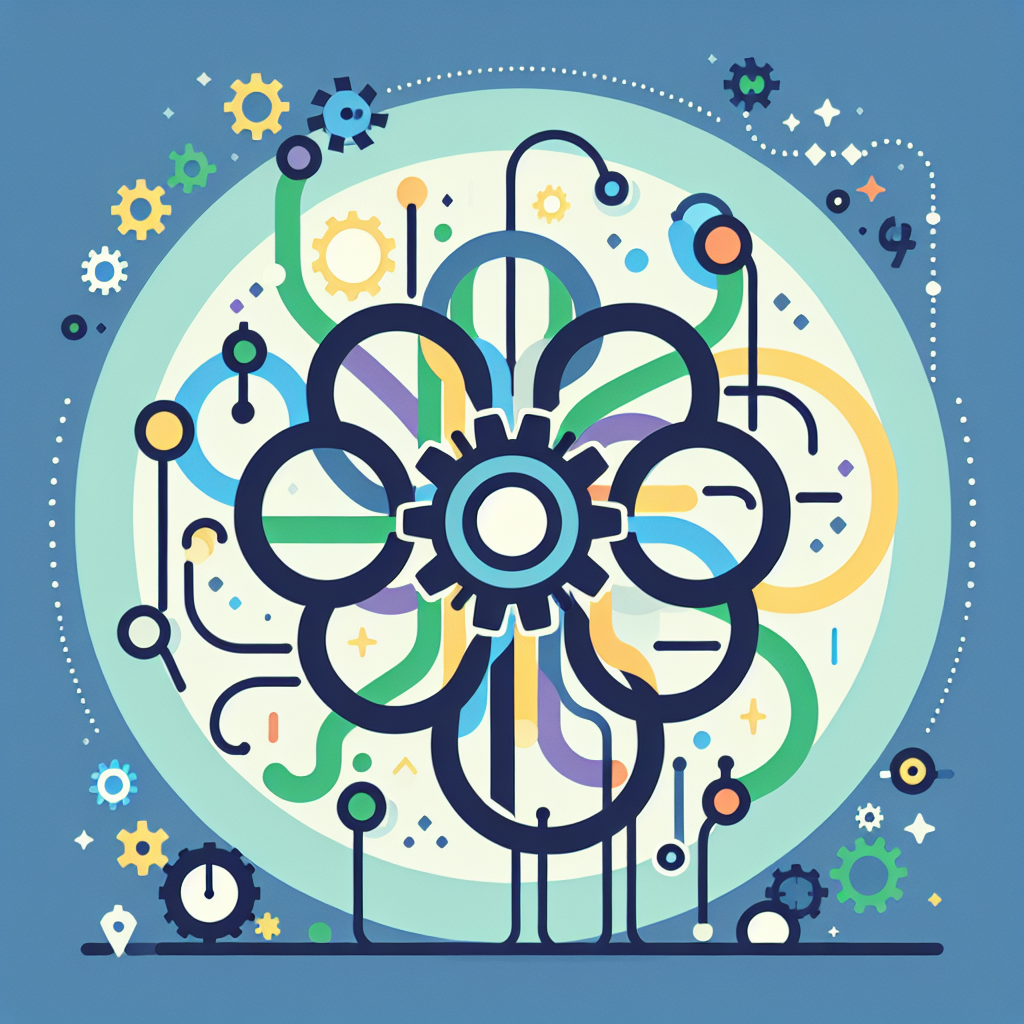
Introduction to Golang Concurrency
Concurrency is a fundamental concept in computer science that allows multiple tasks to be executed simultaneously, improving the efficiency and performance of software applications. In the world of programming, Golang, or Go, stands out for its robust concurrency model, which is designed to make concurrent programming straightforward and efficient. This blog post delves into Golang concurrency, exploring key components like goroutines and Go channels, and how they facilitate parallel execution.
Understanding Golang Concurrency
Golang’s concurrency model is built on the idea of goroutines, lightweight threads managed by the Go runtime. Unlike traditional threads, goroutines are highly efficient in terms of memory and processing power, making them ideal for scalable applications.
What are Goroutines?
Goroutines are functions or methods that run concurrently with other functions or methods. They are incredibly lightweight, with a memory footprint of just a few kilobytes. This efficiency allows developers to launch thousands of goroutines without exhausting system resources.
To start a goroutine, you simply use the go
keyword before a function call:
package main
import (
"fmt"
"time"
)
func printMessage(message string) {
for i := 0; i < 3; i++ {
fmt.Println(message)
time.Sleep(time.Millisecond * 500)
}
}
func main() {
go printMessage("Hello from Goroutine!")
printMessage("Hello from Main!")
}
In this example, printMessage
runs concurrently as a goroutine alongside the main function.
Parallel Execution with Goroutines
Parallel execution refers to the ability of a system to perform multiple operations simultaneously. Golang’s scheduler efficiently maps goroutines to available CPU cores, enabling parallel execution on multicore processors.
Example: Concurrent Web Requests
An excellent illustration of parallel execution is handling multiple web requests concurrently:
package main
import (
"fmt"
"net/http"
"time"
)
func fetchURL(url string) {
start := time.Now()
resp, err := http.Get(url)
if err != nil {
fmt.Println(err)
return
}
defer resp.Body.Close()
fmt.Printf("Fetched %s in %v\n", url, time.Since(start))
}
func main() {
urls := []string{
"https://www.google.com",
"https://www.github.com",
"https://www.golang.org",
}
for _, url := range urls {
go fetchURL(url)
}
time.Sleep(time.Second * 5)
}
This code snippet demonstrates how goroutines can handle multiple URLs simultaneously, reducing the overall time required to fetch data from each source.
Synchronizing with Go Channels
While goroutines enable concurrent execution, coordination and communication between them require Go channels. Channels provide a safe way to send and receive data between goroutines, preventing race conditions and ensuring data integrity.
Basics of Go Channels
Channels in Go are typed conduits that allow goroutines to communicate. To create a channel, use the make
function:
channel := make(chan string)
Sending and Receiving Data
Data can be sent to a channel using the <-
operator. Similarly, you can receive data from a channel:
package main
import (
"fmt"
)
func sendMessage(channel chan string) {
channel <- "Hello, Channel!"
}
func main() {
channel := make(chan string)
go sendMessage(channel)
message := <-channel
fmt.Println(message)
}
Buffered Channels
Buffered channels provide a buffer space, allowing multiple values to be sent without blocking. This can be helpful for optimizing performance in specific scenarios.
package main
import "fmt"
func main() {
channel := make(chan int, 3)
channel <- 1
channel <- 2
channel <- 3
fmt.Println(<-channel)
fmt.Println(<-channel)
fmt.Println(<-channel)
}
In this example, the buffered channel holds three integers, demonstrating how buffered channels can manage data flow efficiently.
Real-World Applications of Golang Concurrency
Golang’s concurrency model is widely used across various industries. For example, in web servers and microservices, handling thousands of requests concurrently becomes seamless with goroutines. According to a Go Developer Survey by the Go team, 75% of developers use Go for web programming, where concurrency plays a pivotal role.
Moreover, financial applications that require real-time data processing benefit from Go’s ability to handle concurrent transactions without compromising performance.
Best Practices for Using Golang Concurrency
- Limit Goroutines: Avoid spawning too many goroutines to prevent overwhelming the system.
- Use Channels Judiciously: Employ channels for communication between goroutines to maintain data integrity.
- Monitor Goroutines: Use Go tools to monitor the number of active goroutines to optimize resource usage.
Conclusion
Golang concurrency, with its goroutines and Go channels, offers a powerful and efficient way to handle concurrent tasks, making it a preferred choice for developers building scalable and high-performance applications. By understanding and leveraging these tools, you can enhance your applications’ responsiveness and throughput, ultimately delivering a superior user experience.