Master Go mod init: Simplify Your Go Projects Now
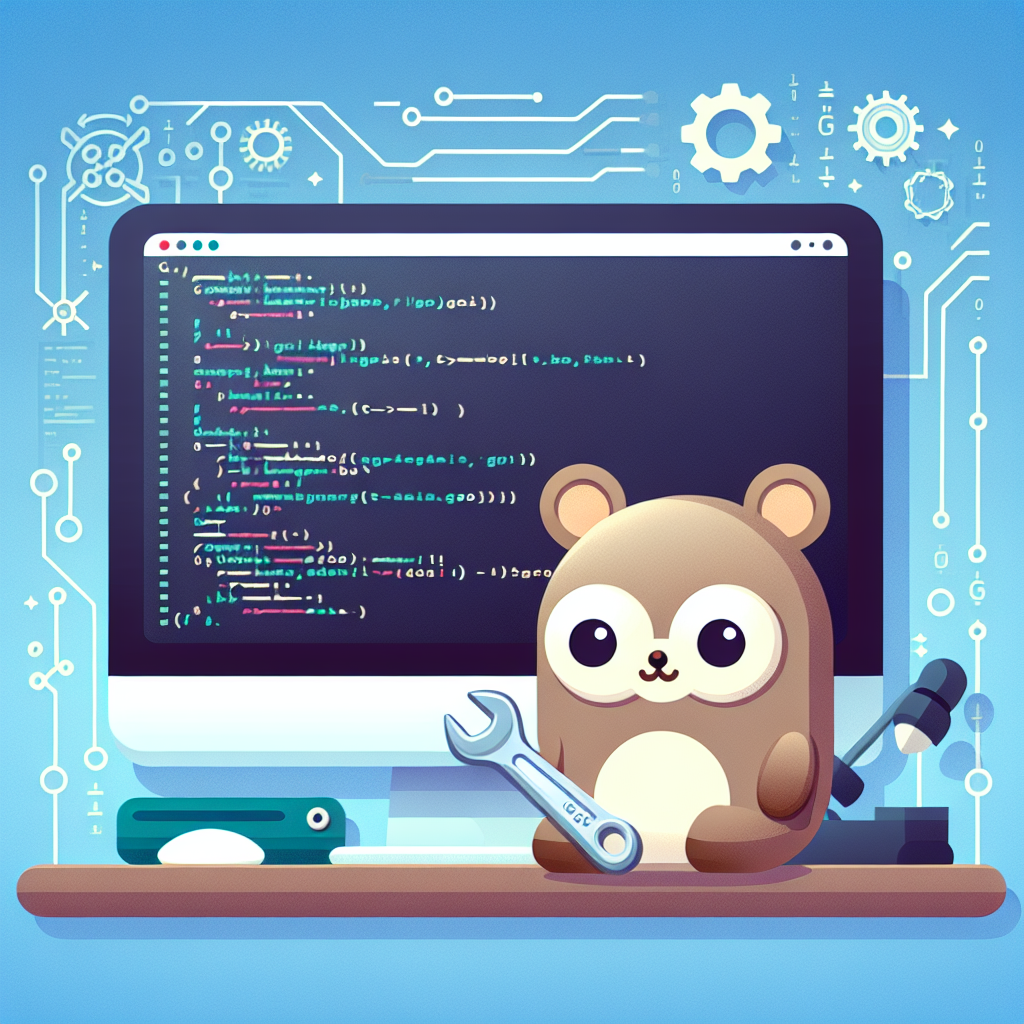
Introduction
In recent years, the Go programming language has gained significant traction in the software development community due to its simplicity, efficiency, and robust concurrency support. One of the key features that make Go appealing is its streamlined approach to dependency management, primarily facilitated through the go mod init
command. This post will explore the mechanics of go mod init
, its role in Go module management, and how it simplifies Go project setup and dependency management.
Understanding Go Modules
Go modules are a dependency management system introduced in Go 1.11 to replace the traditional GOPATH-based workflow. They provide a way to manage project dependencies, versioning, and ensure reliable builds.
Benefits of Using Go Modules
- Versioning: Allows specifying exact versions of dependencies, ensuring consistent builds.
- Isolation: Each project can have its own set of dependencies, removing conflicts.
- Compatibility: Supports backward compatibility with GOPATH.
The Role of go mod init
The go mod init
command is pivotal in creating a new Go module. It initializes a module by creating a go.mod
file in the project root. This file acts as the manifest for your project, listing its dependencies and module path.
How to Use go mod init
To start using Go modules, navigate to your project directory and run the following command:
go mod init <module-path>
- module-path: Typically, this is the repository URL or the desired import path for your module.
Example
Suppose you are developing a new web application hosted on GitHub. You might execute:
go mod init github.com/username/my-web-app
This command creates a go.mod
file similar to:
module github.com/username/my-web-app
go 1.19
Impact of go.mod
- Dependency Management: Lists dependencies with their versions.
- Project Setup: Establishes the module’s root, simplifying project setup.
- Build Consistency: Ensures build reproducibility across environments.
Deep Dive into Go Dependency Management
Effective dependency management is crucial for any software project. Go’s module system automates much of this process, allowing developers to focus more on code and less on configuration.
Adding Dependencies
Once your module is initialized, you can add dependencies using the following command:
go get <package>
For example:
go get github.com/gin-gonic/gin
This command updates the go.mod
file to include the new package and its version.
Updating Dependencies
To update dependencies to their latest versions, use:
go get -u
This ensures that all packages are up-to-date, leveraging the latest features and security patches.
Resolving Dependency Conflicts
Go modules handle dependency conflicts through semantic versioning. The go mod tidy
command helps clean up unused dependencies and resolve potential conflicts:
go mod tidy
This command ensures your go.mod
and go.sum
files are accurate and up-to-date.
Best Practices for Go Project Setup with Modules
To maximize the efficiency of Go module management, consider these best practices:
Consistent Naming Conventions
- Use clear, descriptive module paths.
- Follow standard naming conventions for readability and maintainability.
Regular Dependency Updates
- Regularly update dependencies to benefit from new features and security improvements.
- Always test your application after updates to ensure stability.
Use Semantic Versioning
- Adhere to semantic versioning (e.g., MAJOR.MINOR.PATCH) for your modules.
- Clearly document any breaking changes in version updates.
Conclusion
The go mod init
command is a cornerstone of modern Go development, simplifying project setup and dependency management. By leveraging Go module management, developers can ensure consistent, reliable builds and reduce the complexity associated with dependency management. With these tools and practices, you’ll be well-equipped to create efficient, scalable Go applications. As the Go ecosystem continues to evolve, staying informed about best practices and tools will be key to maintaining robust and maintainable codebases.