Master HTML Escaping: Enhance Web Security Today
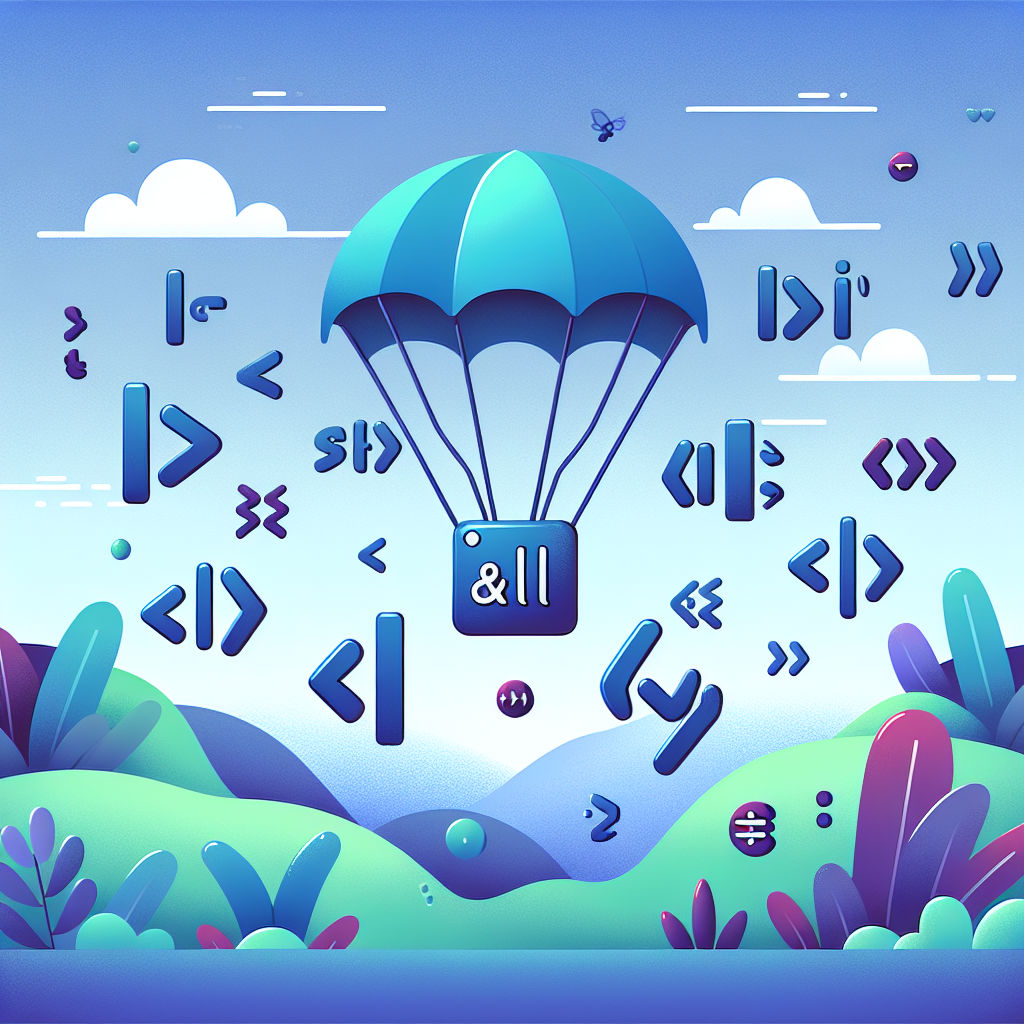
Understanding HTML Escaping Techniques
HTML escaping is a crucial technique in web development that enhances security and functionality. By converting special characters into HTML character entities, developers can prevent security vulnerabilities and ensure proper display in web browsers. This blog post delves into HTML escaping techniques, discussing their importance in secure coding practices and web security.
Why HTML Escaping is Essential
In the world of web development, user input is often unpredictable. Without proper handling, special characters can disrupt HTML structure or even lead to serious security risks like Cross-Site Scripting (XSS). HTML escaping mitigates these risks by converting potentially hazardous characters.
The Basics of HTML Character Entities
HTML character entities are used to display reserved characters in HTML. Here are some common entities:
&
becomes&
<
becomes<
>
becomes>
"
becomes"
'
becomes'
Using these entities ensures that browsers interpret characters as intended, rather than as HTML code.
Implementing HTML Escaping in Your Code
JavaScript HTML Escaping Example
In JavaScript, you can create a function to convert special characters into their respective HTML entities:
function escapeHTML(str) {
return str.replace(/&/g, "&")
.replace(/</g, "<")
.replace(/>/g, ">")
.replace(/"/g, """)
.replace(/'/g, "'");
}
console.log(escapeHTML('<script>alert("XSS!")</script>'));
// Output: <script>alert("XSS!")</script>
This function ensures that user inputs containing special characters are safely escaped.
HTML Escaping in Server-Side Languages
Server-side languages like PHP also offer built-in functions for escaping:
$user_input = '<b>Bold Text</b>';
$safe_input = htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8');
echo $safe_input; // <b>Bold Text</b>
htmlspecialchars()
converts special characters, preventing them from being interpreted as HTML.
Secure Coding Practices with HTML Escaping
Adopting secure coding practices involves consistently escaping user inputs. Here are a few guidelines:
- Escape on Input and Output: Always sanitize inputs when they are received and escape outputs when they are displayed.
- Use Built-in Functions: Leverage built-in functions in programming languages for escaping, as they are optimized for security.
- Stay Updated: Regularly update libraries and frameworks to protect against the latest security threats.
Enhancing Web Security with HTML Escaping
HTML escaping plays a vital role in safeguarding web applications. According to OWASP, XSS attacks constitute a significant portion of security vulnerabilities in web applications. Proper escaping can drastically reduce the risk of such attacks.
Best Practices for HTML Escaping
- Consistent Escaping: Apply escaping consistently across all user inputs.
- Understand Contexts: Different contexts (HTML, JavaScript, URLs) may require different escaping techniques.
- Testing: Regularly test your application for security vulnerabilities, ensuring that escaping techniques are effectively implemented.
Conclusion
HTML escaping techniques are indispensable for developers aiming to create secure and reliable web applications. By converting special characters into HTML character entities and following secure coding practices, developers can enhance web security and ensure that applications perform as expected. Remember, consistent and proper use of escaping techniques is a fundamental step towards building robust web applications and protecting against security threats. Embrace these practices to safeguard your projects and maintain user trust.