Check if a Directory Exists in Python: Quick Guide
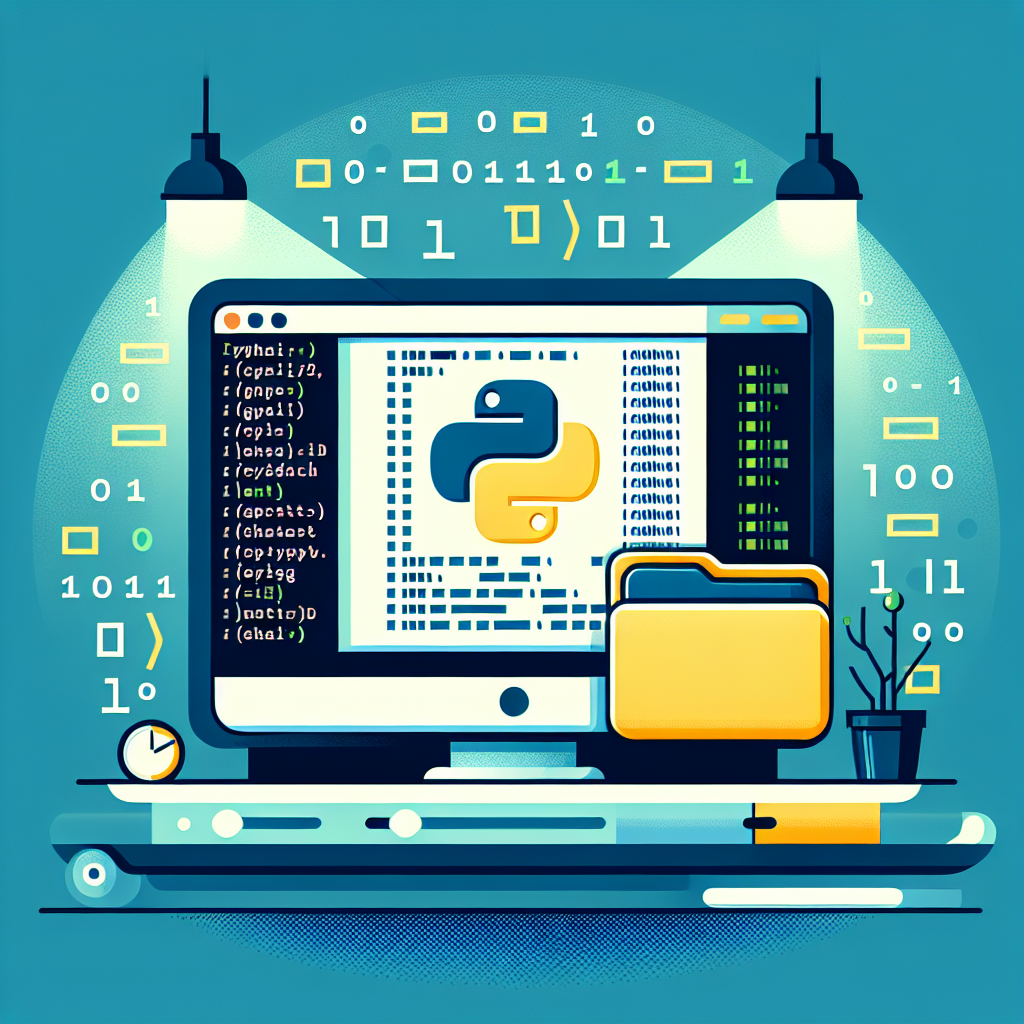
Introduction
In today’s digital age, managing files and directories efficiently is crucial for developers. Whether you’re building a new application or maintaining an existing one, ensuring that your program interacts with the file system correctly is key. One common task is checking if a directory exists in the system before performing operations like reading, writing, or modifying files. In this blog post, we’ll explore how to perform a Python directory check using the os.path.exists
function, among other methods. This guide aims to equip you with the knowledge to handle file system operations seamlessly in your Python projects.
Why Check If a Directory Exists?
Before delving into code, let’s discuss why checking if a directory exists is essential:
- Prevent Errors: Attempting to read from or write to a non-existent directory can lead to runtime errors.
- Resource Management: Ensures resources are allocated correctly and prevents unnecessary operations.
- Data Integrity: Helps maintain data integrity by ensuring files are saved in the intended locations.
Understanding these reasons highlights the importance of performing a directory check before proceeding with any file operations.
Using os.path.exists
for Directory Check
The os
module in Python provides a variety of functions for interacting with the operating system. One of these is os.path.exists
, which can be used to check if a path exists, whether it’s a file or a directory.
Example Code
Here’s a simple example of how to use os.path.exists
to check if a directory exists:
import os
def check_directory_exists(directory_path):
return os.path.exists(directory_path)
## Example usage
directory = "/path/to/directory"
if check_directory_exists(directory):
print("Directory exists!")
else:
print("Directory does not exist.")
Explanation
- Importing the Module: We begin by importing the
os
module. - Function Definition: A function
check_directory_exists
is defined, which takes a directory path as input and returns a boolean indicating the presence of the directory. - Usage: The function is called with a sample directory path. Depending on the existence of the directory, it prints the appropriate message.
Alternatives to os.path.exists
While os.path.exists
is a popular choice, Python offers other ways to perform a directory check. Let’s explore a couple of alternatives.
Using os.path.isdir
The os.path.isdir
function checks specifically for directories, ignoring files. It’s useful when distinguishing between files and directories is necessary.
import os
def is_directory(directory_path):
return os.path.isdir(directory_path)
## Example usage
directory = "/path/to/directory"
if is_directory(directory):
print("This is a directory!")
else:
print("This is not a directory.")
Using pathlib
Introduced in Python 3.4, pathlib
provides an object-oriented approach to file system handling. It’s both powerful and intuitive.
from pathlib import Path
def check_dir_with_pathlib(directory_path):
return Path(directory_path).is_dir()
## Example usage
directory = "/path/to/directory"
if check_dir_with_pathlib(directory):
print("Directory exists using pathlib!")
else:
print("Directory does not exist using pathlib.")
Best Practices for File System Handling
When working with file systems, adhering to best practices ensures efficient and error-free operations.
Use Absolute Paths
Always use absolute paths to avoid ambiguity and ensure your program accesses the correct directory.
Handle Exceptions
Wrap your directory checks with exception handling to manage unexpected issues gracefully.
import os
def safe_check_directory(directory_path):
try:
if os.path.exists(directory_path):
print("Directory exists!")
else:
print("Directory does not exist.")
except Exception as e:
print(f"An error occurred: {e}")
Validate Inputs
Before performing any operations, validate the input to ensure it’s a well-formed path.
Conclusion
Checking if a directory exists is a fundamental task in Python programming, critical for robust file system interactions. Whether you choose os.path.exists
, os.path.isdir
, or pathlib
, Python provides versatile tools to perform these checks effectively. By integrating these techniques into your projects, you can prevent errors, manage resources efficiently, and maintain data integrity. Remember to adhere to best practices like using absolute paths and handling exceptions to create reliable and maintainable code. With this knowledge, you’re well-equipped to handle file system operations in your Python applications confidently.