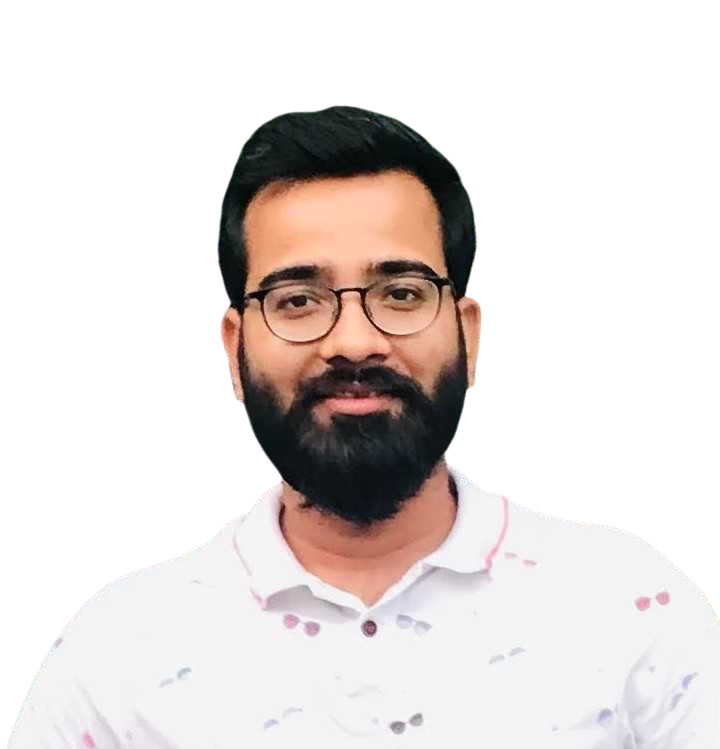
👋 Welcome to my corner of the web
Hey, I'm Kshitij Singh
Programmer – Traveller – Husband – Generalist
I'm a tech geek who loves to write about programming, tech hacks, and platform guides. Building tools that make developers' lives easier.
Skills & Technologies
Frontend
React Vue TypeScript Tailwind
Backend
Node.js Python Go PostgreSQL
DevOps
Docker AWS CI/CD Kubernetes
Tools
Git VS Code Figma Postman
About me
- 🎂 27 Years old
- 🌍 From India
- 💻 Software developer
- 🎮 Part time Indie Hacker
- 🏍️ Loves Bike Trips
Experience
- ZeptoLead Software Engineer (Present)
- GoJekSE2 (2020-2022)
- NokiaR&D Engineer (2018-2020)
Products Launched
0
+3
And counting...
Featured Projects
Swapcode AI
The AI-powered development suite that helps you write, convert, and debug code 10x faster
SuperDMs
Bulk Twitter DMs SAAS platform for efficient communication
Nameless
Anonymous chat messenger for private conversations
Kanbang
Minimal but fancy Kanban board for task management
Ideator
Twitter post idea generator powered by AI
Chootu
A quick and short url shortener